10 Tips for Success with Typescript Unit Testing
Have you ever run a unit test that failed the first time but magically passed on a second try? Or spent forever debugging, only to discover the actual bug was in the test itself? These headaches are typical in TypeScript unit testing—often stemming from type mismatches, flaky tests, or sluggish execution times.
According to GitLab’s DevSecOps report, about 70% of teams now automate unit tests. Besides catching bugs early, well-structured unit tests boost an application’s maintainability, debugging speed, and overall scalability.
Below, you’ll find 10 key strategies to help you build robust TypeScript unit tests that keep your code reliable and easy to update.
TypeScript’s static typing helps eliminate many runtime errors but cannot catch every logical bug. Here, unit tests step in. They illuminate issues that type definitions alone cannot prevent.
Below are three core reasons why TypeScript unit testing matters so much.
1. Ensures Type Safety in Tests
TypeScript’s type system effectively identifies mismatches at compile time but doesn’t ensure that functions behave as intended. A function may return the correct type yet still have logical errors.
For example, the below test checks whether the calculatePrice function correctly applies a percentage discount to ensure it returns the expected value.

Code snippet demonstrating a function applying a discount to illustrate unit tests ensuring type safety.
2. Prevents Regressions and Improves Maintainability
When refactoring a TypeScript code, the compiler might not catch logical changes that break functionality. For example, if a function’s signature or behavior is modified during a refactor (return type number to string), tests can immediately reveal discrepancies even when TypeScript reports no type errors.
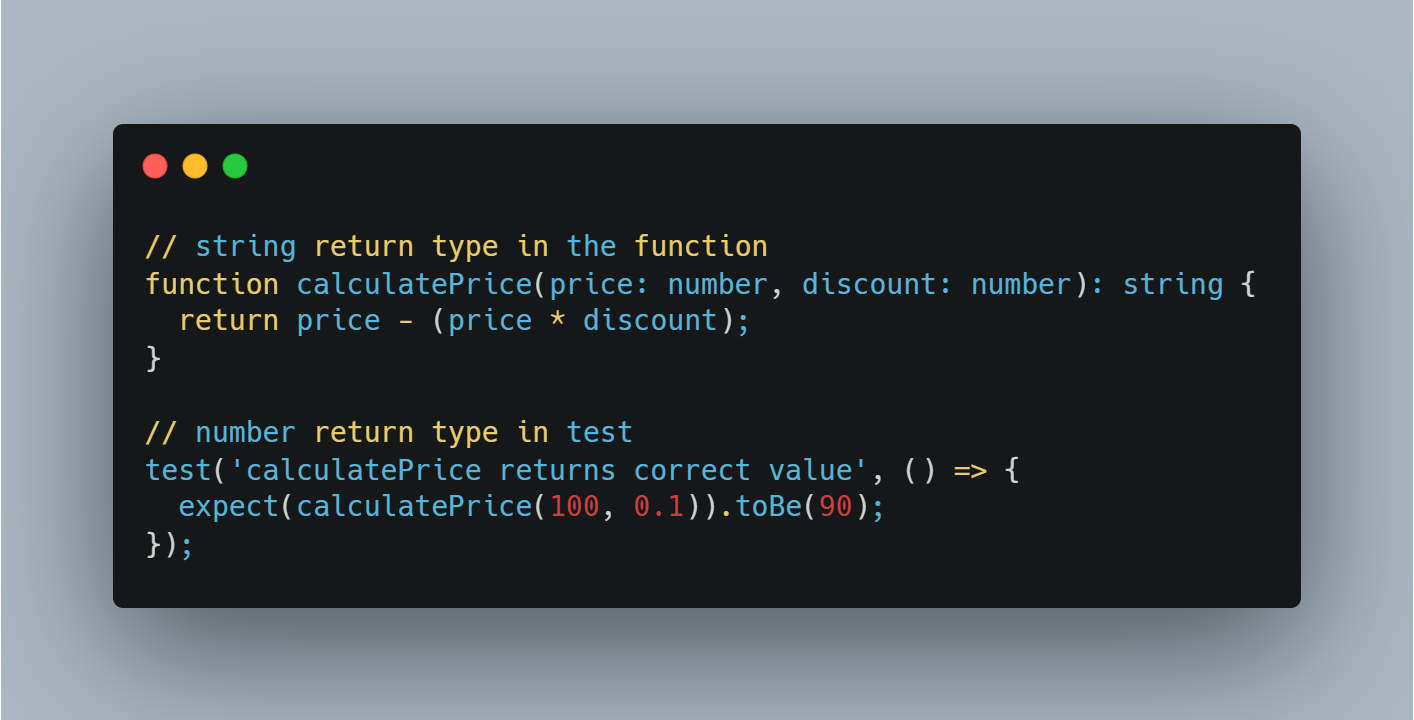
Example function showing TypeScript return types to verify expected return values in tests.
3. Enables Faster Debugging and Development Cycles
A solid test suite gives you immediate clarity when something breaks. When you run your tests, you’ll know which function or module is failing—and why—so you can skip the endless logs or guesswork. Tracking developer productivity metrics, such as cycle time and defect rate, can provide further insights into how effectively debugging and testing processes are improving over time.
Test-Driven Development (TDD) is worth a try if you want even more structure. However, in practice, many teams use TDD inconsistently—or just enough to say they tried. This is how tests are often implemented.
- Write your test first.
- Watch it fail (as expected).
- Implement just enough code to make that test pass.
The real point of TDD goes beyond passing tests: it’s about preventing long-term technical debt before it piles up. When you write tests first, you naturally design more modular code and catch potential pitfalls early, ultimately saving time (and headaches) further into the project.
Here are 10 tips for getting your TypeScript unit testing right.

Unit test for division by zero, demonstrating how error handling prevents regressions in refactored code.

Selecting your framework—whether it’s Jest, Mocha, Vitest, or AVA—is the foundation of your testing approach. Each option has a different philosophy for handling mocks, assertions, and asynchronous tests, so pick one that best suits your codebase size, project goals, and team experience.
Lean, highly concurrent testing for simplicity and speed
But picking the right framework is just the first hurdle. The real work comes when you must maintain test coverage, manage stubs, and keep all those specs up-to-date every time your code changes.
Advanced automation can be a lifesaver when your codebase grows. A typical coverage tool might show you which lines need testing. Still, an AI-driven testing agent (like EarlyAI) takes it further by scanning your source code, adapting to your chosen framework, and calling out incomplete or outdated tests before they cause real trouble.
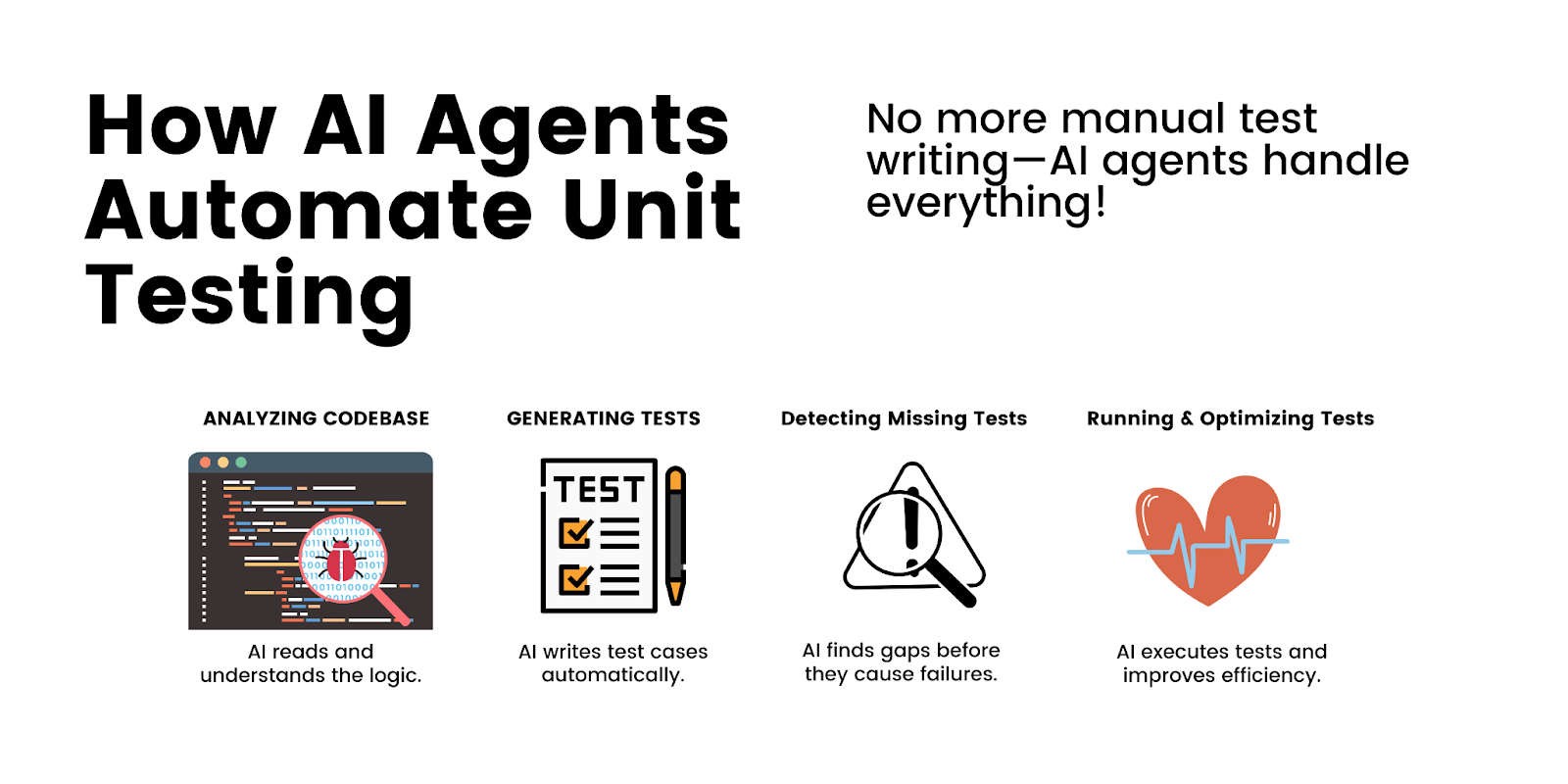
Diagram highlighting AI-driven unit testing automation, explaining how AI can streamline test writing and maintenance.
Getting your TypeScript configuration in order is the backbone of a seamless test environment. To achieve more efficient builds, enable strict mode in your tsconfig.json, include all relevant directories, and consider flags like esModuleInterop and skipLibCheck.
A well-tuned tsconfig.json prevents type-related headaches and gives your tests a more stable foundation, saving you from time-consuming refactors later.
Once you’ve nailed the compiler settings, you need to think about how and where to arrange your tests so they’re easy to maintain, debug, and scale. Good test structure—such as clear naming conventions, logical folder organization, and consistent patterns for unit vs. integration tests—can save you from hours of confusion later. Unit testing best practices exist to prevent this confusion from happening.
Mocking is essential for keeping your tests isolated from outside dependencies. Libraries like Jest provide methods like jest.mock() to mock modules or fake API calls so that tests remain isolated and reliable. However, integrating automated penetration testing alongside unit tests for teams working with security-sensitive applications ensures vulnerabilities aren't overlooked in CI/CD workflows.
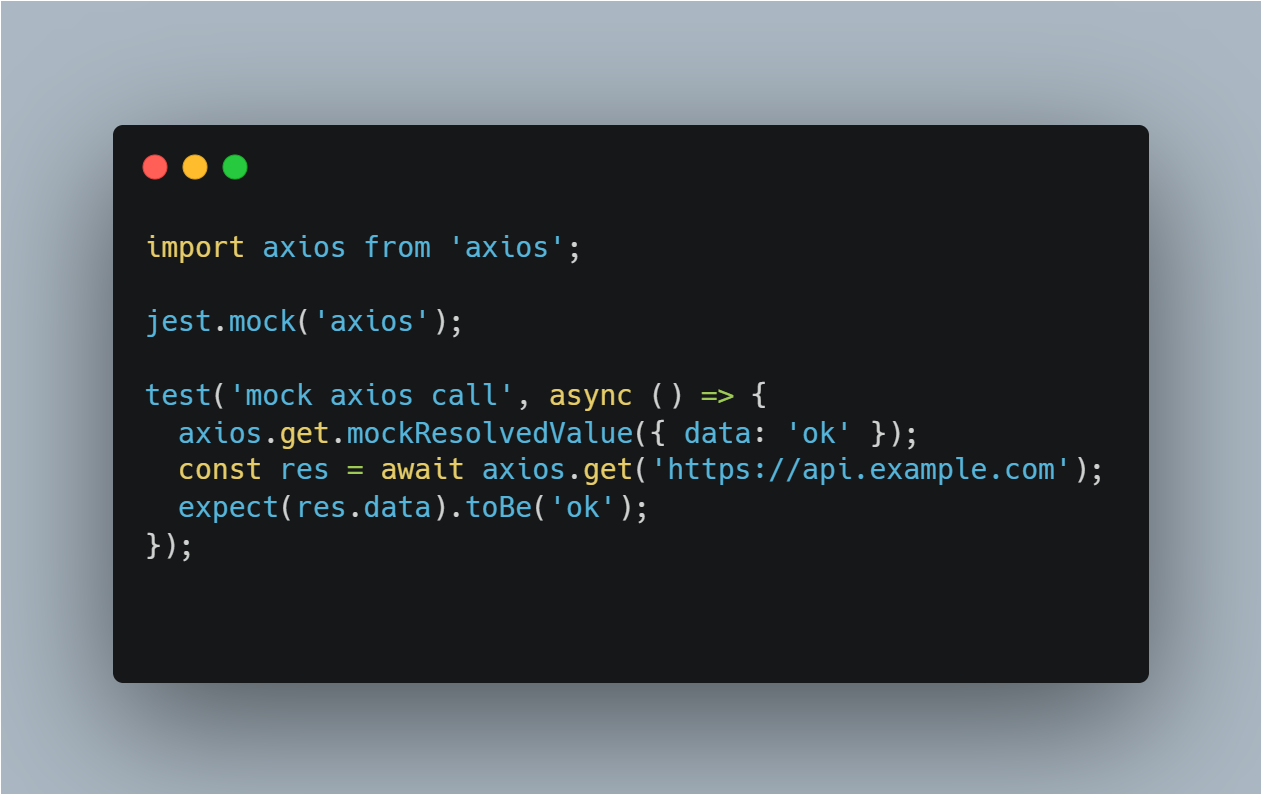
Code snippet using async functions, demonstrating proper handling of asynchronous test cases.
When you mock external services, you remove the risk of a failing test caused by an offline API or unpredictable network lag. Treating those mocks with the same level of scrutiny as production code is essential—poorly secured stubs or configurations can create attack vectors in your CI/CD pipeline.
Use explicit type definitions to clarify your test inputs and outputs. When your functions declare exactly what data shapes they expect and return, TypeScript flags mismatches immediately—no surprise runtime failures. This approach also makes your test cases more straightforward to maintain since future contributors can instantly see which properties or data structures your code should be handling.
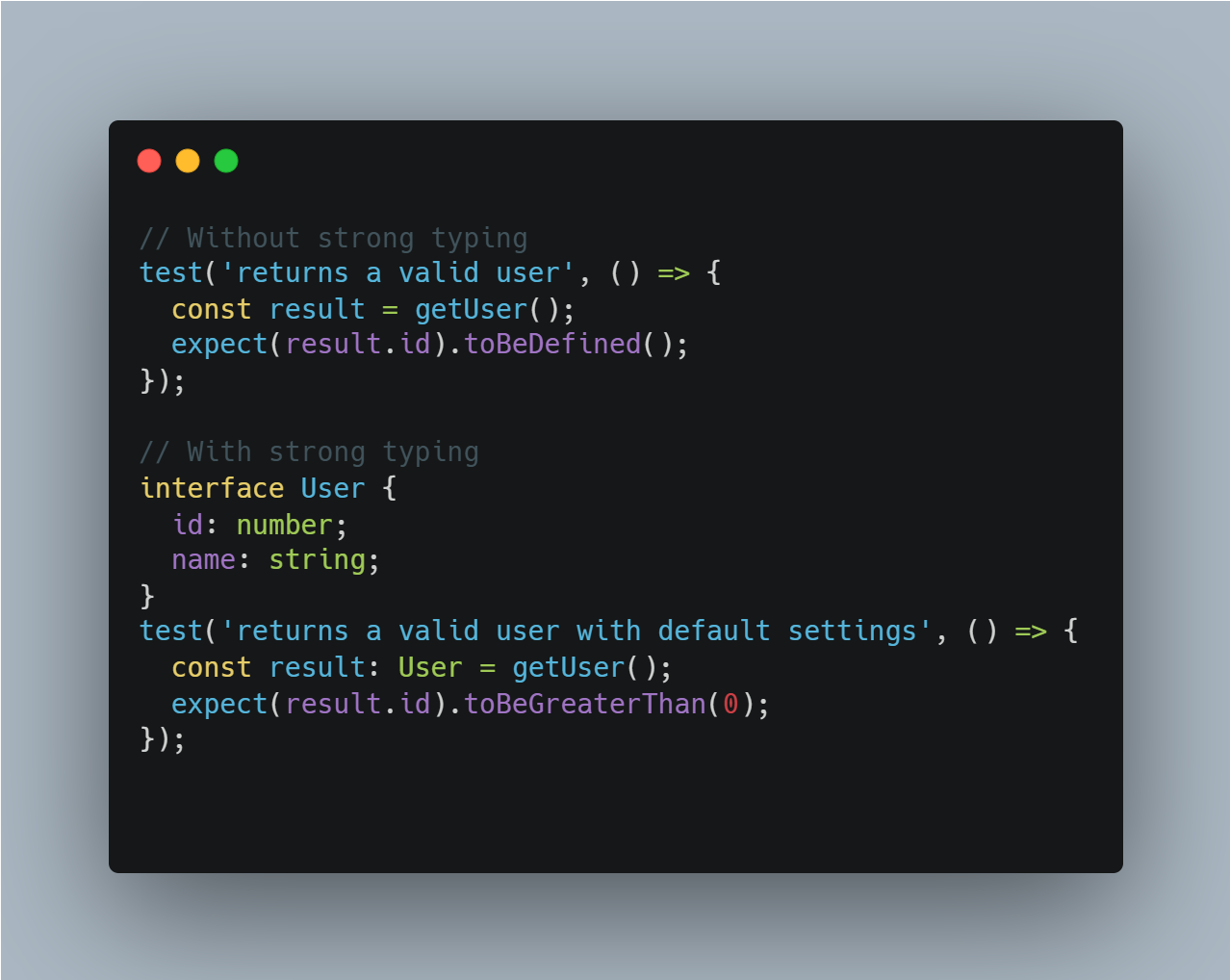
Unit test using TypeScript interfaces to validate object properties and expected data structures.
Give each test a descriptive name that pinpoints exactly what you’re testing and why. A clear, specific name helps you spot failures faster and lowers the learning curve for new contributors. When someone sees “should_apply_discount_for_premium_users,” they immediately know the scenario—and what’s supposed to happen.
If you’re new to Jest or any other testing framework, check out a developer-friendly guide to ensure your test titles, setup, and teardown steps are consistent and logical.

Example test function verifying object properties.
Don’t just test the happy path—focus on everything that can go wrong.
- Null and Undefined Inputs: Validate your code's behavior when given missing or empty values.
- Exception Handling: Confirm that your functions throw or handle errors in predictable ways.
- Boundary Conditions: Check performance and correctness when numbers or string lengths approach certain limits.
Beyond typical edge cases, modern applications must also account for security vulnerabilities in their test suites. For example, failing to validate and sanitize inputs properly can lead to remote code execution (RCE) exploits, where attackers inject and execute malicious code within your application. Understanding these vulnerabilities and how they manifest is essential for writing robust test cases that prevent such threats before they reach production.
Taking it further, AI agents can automatically generate tests for corner cases by scanning your function signatures and spotting areas where untested logic might hide. Let the AI handle edge-case detection so you can concentrate on bigger architectural decisions instead of manually discarding weird input.
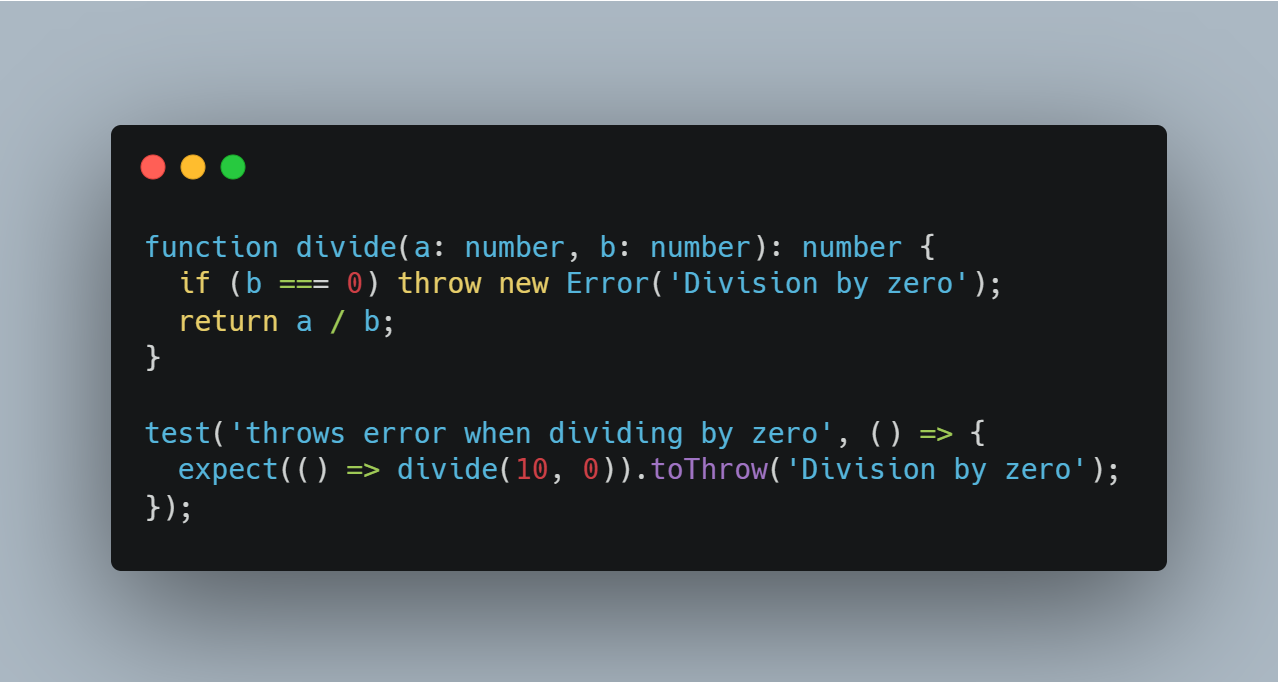
Example of a test case verifying that an error is thrown for division by zero, ensuring exception handling is properly tested.
Optimizing test performance in large codebases is crucial to maintain efficiency and rapid development cycles. When projects scale up, even a single test run can grind your entire pipeline to a halt. To avoid this, split tests across multiple cores are enabled by parallel execution (for example, jest --maxWorkers), so each CPU core handles its share of the load to cut run times drastically on multi-core systems.
Keeping every test file self-contained is also crucial—any shared state or hidden dependencies can introduce race conditions. If you have a massive repository, consider segmenting your tests by feature or module and running them selectively based on what changed in the last commit (e.g., using watch mode) to reduce the workload dramatically so you’re not re-testing unrelated parts of your codebase.
Finally, cache or save your test results when possible. Many CI systems can skip re-checking unchanged components, making your pipeline leaner and faster with every subsequent commit.

Terminal screenshot showing Jest test execution output, including a summary of passing and failing tests.
Organize your test files with a consistent naming scheme, and keep unit, integration, and end-to-end tests in their folders. That way, there’s no confusion about scope, and both new developers and automated tooling can locate tests quickly. A structured approach also reduces coverage gaps and keeps everyone on the same page as your codebase expands.

File structure diagram illustrating best practices for organizing unit and integration tests in a TypeScript project.
Group your tests by feature or module so it’s always clear what’s tested and why. At the same time, employ smart mocking strategies—like partial stubs or module-level overrides—to isolate external dependencies without losing sight of real-world behavior. This dual approach keeps tests straightforward while reflecting genuine application flows, especially if your system relies on microservices or multiple components.
Coverage reports illuminate which parts of your code remain untested—down to the function or line. Tools like Istanbul (built into Jest) provide a clear breakdown, and if you pair those insights with an AI-driven solution, you can pinpoint every missed path automatically.
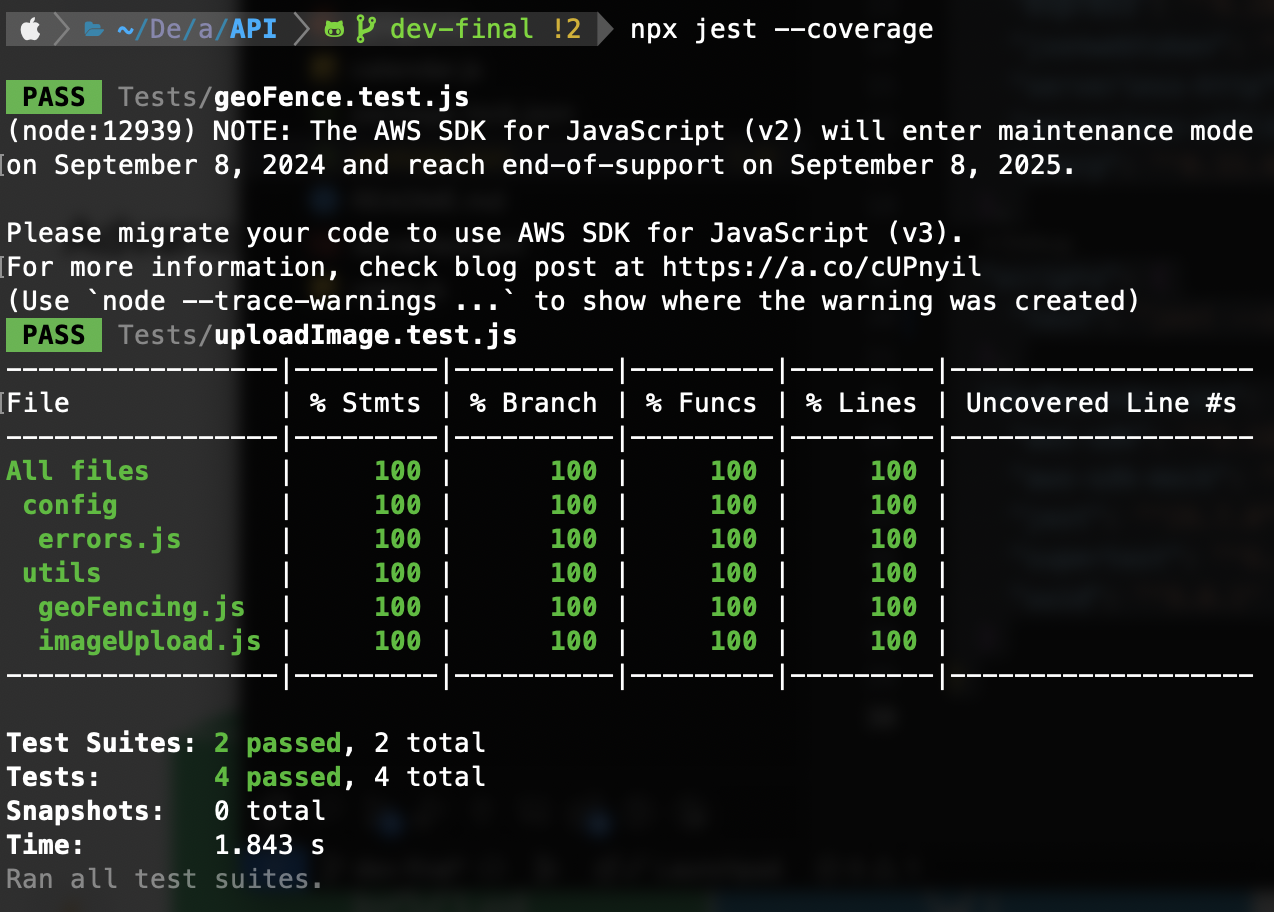
Terminal screenshot running Jest with --coverage, showing code coverage metrics to ensure test completeness.
AI assistants provide one-off suggestions or code snippets. Agentic AI solutions actively manage your entire testing process in real-time. Here are the main things they can do:
- monitor your codebase for new or modified logic
- dynamically adapt coverage strategies, automatically generating or updating tests as functions evolve
For example, EarlyAI goes beyond simple automation. It handles partial method refactors, watches untested paths, and keeps your test suite in sync without waiting for a human to intervene. This agentic approach continuously aligns your tests with your evolving codebase, eliminating coverage gaps and freeing you to focus on advancing new features instead of patching old tests.
Unit testing with TypeScript is an ongoing strategy to keep your project stable and easy to evolve.
AI agents like EarlyAI fully take over the repetitive aspects of testing, eliminating manual effort so developers can focus on business requirements. If you want to scale your unit testing workflow efficiently, integrate AI into your process using EarlyAI.